How to Prepare for Programming Exams and Excel in the Exam Hall
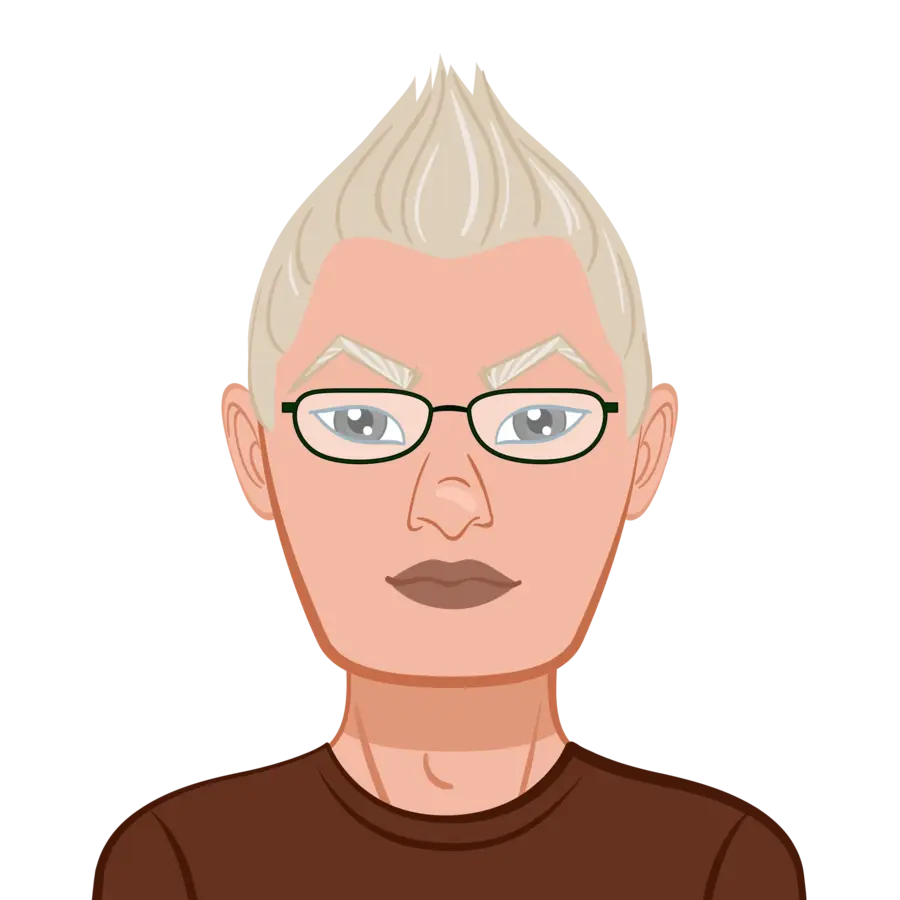
Programming exams require a blend of theoretical knowledge, practical problem-solving skills, and effective time management. These assessments challenge students to think critically, write efficient code, debug errors, and apply logic under pressure. Whether you are preparing for a university-level programming test, an online coding challenge, or a professional certification exam, having a well-structured study plan can significantly boost your performance. Many students struggle with managing complex algorithms, debugging under time constraints, and understanding intricate programming concepts, which is why the right preparation is crucial. If you are wondering, how can I efficiently complete for my programming exam? or seeking an online exam helper to guide you through the toughest programming questions, this guide is here to help. For those considering expert assistance, services that allow students to request professionals to take my programming exam can also be an option. This comprehensive guide will walk you through essential preparation techniques, key areas to focus on, and practical strategies to tackle programming questions confidently in the exam hall.
Understanding the Nature of Programming Exams
Programming exams can vary in structure, but they generally consist of the following types of questions:
- Theoretical Questions – Cover programming concepts, syntax rules, and language fundamentals.
- Coding Challenges – Require writing and executing programs to solve given problems.
- Debugging Tasks – Involve identifying and correcting errors in pre-written code.
- Multiple-Choice Questions (MCQs) – Test conceptual clarity and understanding of programming principles.
Key Topics to Focus On
- Data Structures and Algorithms
- Understanding fundamental data structures like arrays, linked lists, stacks, queues, trees, and graphs.
- Mastering common algorithms such as sorting, searching, and recursion.
- Learning about time and space complexity to write optimized code.
- Programming Language Fundamentals
- Strengthening knowledge of variables, loops, functions, and object-oriented programming (OOP).
- Handling input/output operations and exception handling effectively.
- Utilizing standard libraries and built-in functions efficiently.
- Problem-Solving Techniques
- Developing logical thinking to break down complex problems into simpler components.
- Writing pseudocode and flowcharts before implementing the actual code.
- Using debugging strategies to identify and fix errors quickly.
Effective Strategies for Preparing for Programming Exams
1. Strengthen Your Theoretical and Practical Knowledge
- Master Fundamental Programming Concepts
- Understand syntax rules and error handling techniques.
- Learn how different data types and memory management work.
- Get comfortable with functions, recursion, and OOP principles.
- Solve Coding Problems Regularly
- Beginner, intermediate, and advanced problems on platforms like LeetCode, CodeChef, and HackerRank.
- Implementing different approaches to the same problem to improve efficiency.
- Understanding how real-world problems can be translated into programming logic.
- Analyze Previous Exam Questions
- Recognizing frequently asked question types.
- Understanding time constraints and expected solutions.
- Developing strategies to approach different types of questions effectively.
A solid foundation in programming basics is essential. Ensure that you:
Consistent practice is key to improving problem-solving skills. Work on:
Studying past programming exams helps in:
2. Master Time Management Techniques
- Practice Under Timed Conditions
- Improve speed and accuracy in solving coding challenges.
- Develop the ability to complete theoretical and practical questions within the allotted time.
- Identify areas where you spend excessive time and optimize your approach.
- Prioritize Questions Wisely
- Start with the easiest questions to gain confidence and secure marks quickly.
- Allocate more time for coding and debugging tasks that require deeper thought.
- Avoid getting stuck on one question for too long—move on and return later if time permits.
Simulating the actual exam environment helps you:
Managing your time wisely is crucial during the exam. Follow this approach:
3. Improve Debugging and Optimization Skills
- Learn to Identify Common Errors
- Syntax errors due to incorrect formatting or missing elements.
- Runtime errors caused by division by zero or accessing invalid memory.
- Logical errors leading to incorrect program output.
- Use Debugging Tools Efficiently
- Breakpoints and step-through execution.
- Logging and print statements to check variable values.
- Automated testing to validate code behavior.
Common programming errors include:
Modern IDEs provide powerful debugging features that can help identify problems quickly. Some useful tools include:
Strategies for Handling Programming Questions in the Exam Hall
- Read the Questions Carefully
- Read the problem statement twice to ensure you grasp all requirements.
- Identify constraints like input size, time limits, and expected output format.
- Highlight key details and write down important points before coding.
- Plan Your Solution Before Writing Code
- Break down the problem into smaller steps.
- Decide on the most efficient algorithm to use.
- Write pseudocode or a rough structure before implementing the final code.
- Write Clean, Readable, and Optimized Code
- Using meaningful variable and function names.
- Keeping code modular by using functions instead of long blocks of code.
- Avoiding unnecessary computations and optimizing loops.
- Test Your Code Before Submission
- Run test cases on sample inputs provided in the question.
- Check edge cases and boundary conditions.
- Verify that your program runs within the expected time constraints.
One of the biggest mistakes students make is rushing into solving the problem without fully understanding the question. To avoid this:
Taking a moment to plan can save valuable time. Follow these steps:
Your code should be well-structured and easy to understand. Best practices include:
Before finalizing your solution, ensure that you:
Conclusion
Preparing for programming exams requires a balanced approach that includes theoretical understanding, practical problem-solving, and time management skills. By consistently practicing coding problems, strengthening debugging techniques, and applying strategic approaches in the exam hall, students can significantly enhance their performance. Effective preparation not only helps in securing high scores but also builds confidence in tackling real-world coding challenges. Additionally, learning how to write efficient, optimized code under time constraints is a valuable skill that extends beyond exams and into professional settings. By following structured study methods, staying disciplined, and continuously improving, students can transform their exam preparation into an opportunity for long-term success in programming.